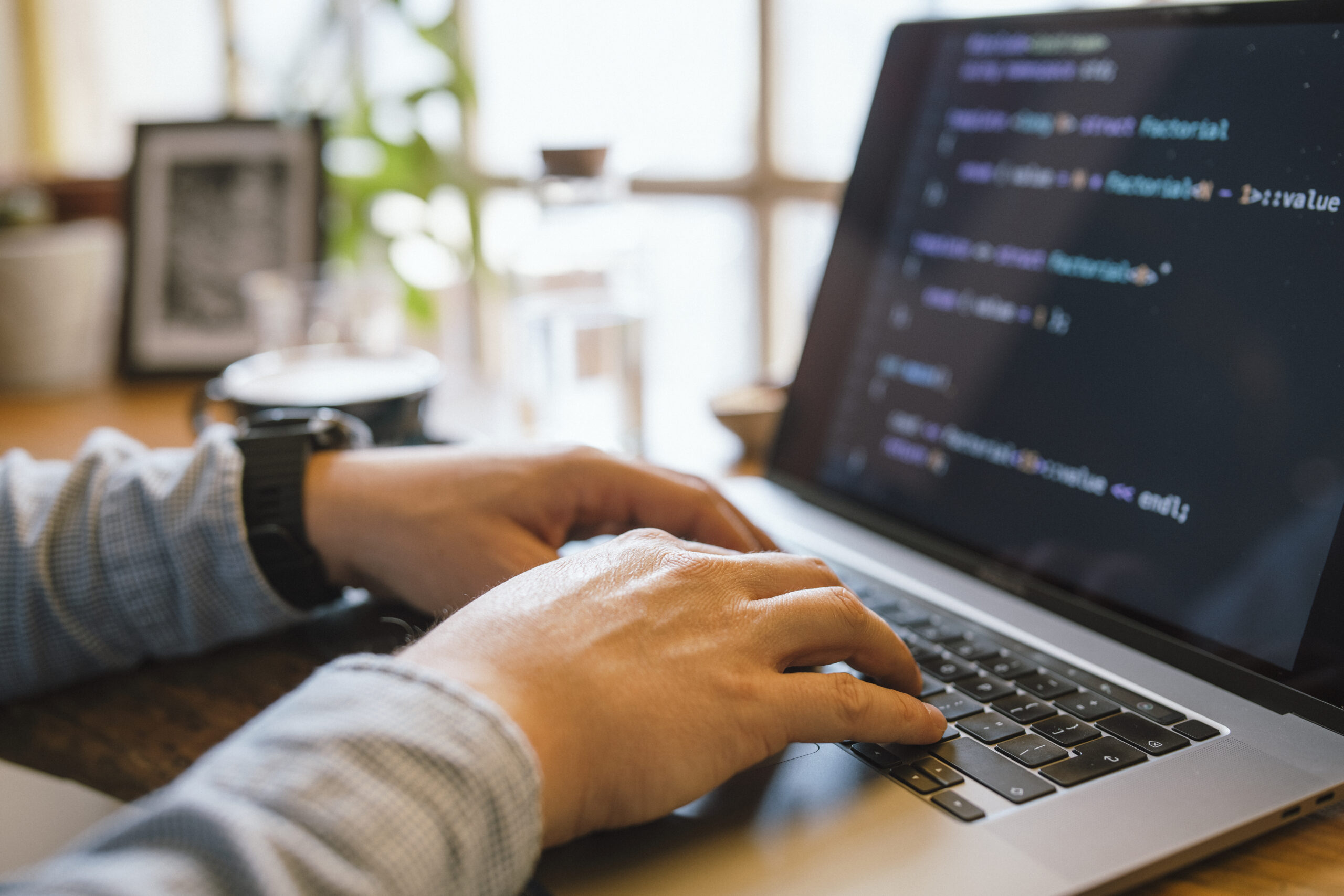
Debugging is Just about the most necessary — yet frequently disregarded — capabilities in a very developer’s toolkit. It isn't really just about fixing damaged code; it’s about comprehending how and why items go Improper, and Finding out to Consider methodically to resolve troubles successfully. No matter if you are a rookie or maybe a seasoned developer, sharpening your debugging capabilities can help save hrs of disappointment and drastically boost your productivity. Listed below are a number of methods to assist builders stage up their debugging match by me, Gustavo Woltmann.
Grasp Your Resources
One of the fastest strategies developers can elevate their debugging abilities is by mastering the tools they use everyday. When composing code is a single A part of development, recognizing tips on how to communicate with it effectively for the duration of execution is equally important. Modern-day advancement environments come Geared up with impressive debugging abilities — but numerous builders only scratch the surface area of what these applications can do.
Just take, for instance, an Built-in Advancement Environment (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These equipment help you set breakpoints, inspect the worth of variables at runtime, action via code line by line, and even modify code to the fly. When applied accurately, they let you notice precisely how your code behaves through execution, that's invaluable for tracking down elusive bugs.
Browser developer applications, including Chrome DevTools, are indispensable for entrance-stop builders. They let you inspect the DOM, check network requests, look at genuine-time general performance metrics, and debug JavaScript inside the browser. Mastering the console, resources, and community tabs can convert disheartening UI troubles into workable tasks.
For backend or technique-degree builders, tools like GDB (GNU Debugger), Valgrind, or LLDB offer you deep Command above jogging procedures and memory administration. Learning these resources could have a steeper Discovering curve but pays off when debugging overall performance troubles, memory leaks, or segmentation faults.
Further than your IDE or debugger, turn into snug with version Handle programs like Git to understand code background, come across the precise instant bugs were introduced, and isolate problematic modifications.
Eventually, mastering your equipment means going past default options and shortcuts — it’s about building an intimate understanding of your growth atmosphere to ensure that when concerns come up, you’re not misplaced at nighttime. The higher you are aware of your applications, the greater time you may shell out resolving the particular difficulty rather then fumbling as a result of the procedure.
Reproduce the situation
Among the most important — and sometimes disregarded — techniques in powerful debugging is reproducing the challenge. Just before jumping into the code or earning guesses, builders will need to make a steady atmosphere or scenario wherever the bug reliably appears. With out reproducibility, fixing a bug becomes a activity of probability, typically leading to squandered time and fragile code alterations.
Step one in reproducing a problem is accumulating as much context as possible. Check with queries like: What steps led to The problem? Which atmosphere was it in — enhancement, staging, or creation? Are there any logs, screenshots, or error messages? The greater detail you may have, the much easier it turns into to isolate the precise situations less than which the bug happens.
Once you’ve gathered adequate information, endeavor to recreate the issue in your neighborhood atmosphere. This might mean inputting the same knowledge, simulating similar consumer interactions, or mimicking system states. If The problem seems intermittently, consider crafting automated assessments that replicate the sting instances or condition transitions associated. These exams not simply aid expose the condition but additionally avert regressions Down the road.
Occasionally, The problem may very well be setting-unique — it might take place only on selected operating methods, browsers, or beneath specific configurations. Employing instruments like Digital machines, containerization (e.g., Docker), or cross-browser testing platforms could be instrumental in replicating these kinds of bugs.
Reproducing the condition isn’t only a phase — it’s a way of thinking. It requires patience, observation, along with a methodical technique. But as soon as you can continually recreate the bug, you're presently midway to correcting it. With a reproducible scenario, You should use your debugging resources extra correctly, exam potential fixes safely, and connect more Evidently with your group or consumers. It turns an abstract complaint into a concrete obstacle — Which’s wherever builders prosper.
Examine and Recognize the Error Messages
Error messages tend to be the most respected clues a developer has when something goes Improper. Instead of seeing them as frustrating interruptions, builders need to find out to treat mistake messages as immediate communications with the technique. They usually tell you exactly what transpired, the place it happened, and at times even why it happened — if you understand how to interpret them.
Commence by studying the information meticulously and in whole. Lots of developers, especially when underneath time strain, look at the primary line and instantly get started earning assumptions. But deeper in the mistake stack or logs might lie the legitimate root result in. Don’t just duplicate and paste error messages into engines like google — study and have an understanding of them very first.
Crack the error down into pieces. Is it a syntax mistake, a runtime exception, or a logic mistake? Does it position to a specific file and line variety? What module or function induced it? These thoughts can guidebook your investigation and stage you towards the responsible code.
It’s also beneficial to be familiar with the terminology in the programming language or framework you’re applying. Error messages in languages like Python, JavaScript, or Java typically stick to predictable styles, and learning to recognize these can considerably speed up your debugging method.
Some glitches are vague or generic, and in Those people instances, it’s critical to look at the context in which the error transpired. Test related log entries, enter values, and up to date changes inside the codebase.
Don’t forget about compiler or linter warnings possibly. These often precede bigger troubles and supply hints about possible bugs.
Eventually, error messages are certainly not your enemies—they’re your guides. Discovering to interpret them correctly turns chaos into clarity, assisting you pinpoint concerns more rapidly, reduce debugging time, and become a much more effective and assured developer.
Use Logging Properly
Logging is The most highly effective applications inside of a developer’s debugging toolkit. When employed properly, it provides true-time insights into how an application behaves, aiding you realize what’s taking place beneath the hood with no need to pause execution or action from the code line by line.
A fantastic logging approach commences with being aware of what to log and at what degree. Frequent logging ranges consist of DEBUG, Data, WARN, ERROR, and Lethal. Use DEBUG for specific diagnostic facts for the duration of growth, Information for common activities (like productive begin-ups), Alert for probable troubles that don’t break the applying, Mistake for true troubles, and FATAL in the event the process can’t keep on.
Steer clear of flooding your logs with excessive or irrelevant facts. Excessive logging can obscure essential messages and slow down your procedure. Target crucial events, condition modifications, enter/output values, and demanding decision points as part of your code.
Format your log messages Evidently and constantly. Include context, for instance timestamps, request IDs, and performance names, so it’s easier to trace issues in distributed systems or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
Through debugging, logs let you observe how variables evolve, what circumstances are fulfilled, and what branches of logic are executed—all without halting This system. They’re Specially valuable in creation environments where by stepping by means of code isn’t probable.
In addition, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that assistance log rotation, filtering, and integration with checking dashboards.
In the end, smart logging is about equilibrium and clarity. Having a very well-thought-out logging strategy, you may reduce the time it will take to identify problems, achieve further visibility into your applications, and improve the Total maintainability and trustworthiness of your code.
Believe Just like a Detective
Debugging is not just a technical activity—it is a method of investigation. To effectively determine and correct bugs, builders ought to approach the method just like a detective fixing a secret. This mentality helps break down sophisticated troubles into workable sections and adhere to clues logically to uncover the root result in.
Commence by collecting evidence. Consider the indicators of the challenge: mistake messages, incorrect output, or effectiveness problems. Much like a detective surveys a crime scene, collect as much relevant information as you are able to with out jumping to conclusions. Use logs, test circumstances, and user reviews to piece together a get more info clear photograph of what’s occurring.
Upcoming, kind hypotheses. Question oneself: What may very well be resulting in this habits? Have any adjustments not too long ago been produced to the codebase? Has this difficulty happened ahead of below comparable conditions? The objective is to slender down opportunities and recognize potential culprits.
Then, exam your theories systematically. Try and recreate the trouble in a managed natural environment. In case you suspect a specific functionality or element, isolate it and verify if The problem persists. Like a detective conducting interviews, talk to your code inquiries and let the outcome lead you nearer to the truth.
Pay back near attention to smaller particulars. Bugs normally cover in the least predicted places—just like a missing semicolon, an off-by-one particular error, or possibly a race condition. Be extensive and patient, resisting the urge to patch The problem without having fully comprehension it. Temporary fixes may possibly disguise the real challenge, only for it to resurface later on.
Last of all, maintain notes on what you experimented with and learned. Just as detectives log their investigations, documenting your debugging course of action can save time for potential difficulties and assist Other folks have an understanding of your reasoning.
By considering similar to a detective, developers can sharpen their analytical expertise, tactic problems methodically, and turn out to be simpler at uncovering concealed challenges in complicated programs.
Produce Checks
Composing assessments is among the simplest ways to boost your debugging capabilities and Over-all enhancement efficiency. Tests not just aid capture bugs early but will also function a security Web that offers you confidence when creating adjustments in your codebase. A properly-examined application is much easier to debug mainly because it helps you to pinpoint exactly where and when a problem takes place.
Get started with device assessments, which center on particular person capabilities or modules. These small, isolated checks can immediately expose irrespective of whether a selected bit of logic is Doing work as anticipated. Whenever a check fails, you instantly know where to search, substantially decreasing the time used debugging. Device exams are Particularly useful for catching regression bugs—challenges that reappear just after Earlier getting mounted.
Subsequent, combine integration assessments and stop-to-finish checks into your workflow. These enable make certain that numerous aspects of your application function alongside one another smoothly. They’re specially beneficial for catching bugs that happen in elaborate programs with numerous factors or providers interacting. If something breaks, your assessments can tell you which Element of the pipeline failed and below what disorders.
Composing tests also forces you to definitely think critically regarding your code. To check a attribute properly, you require to know its inputs, envisioned outputs, and edge instances. This degree of knowledge By natural means potential customers to better code framework and much less bugs.
When debugging a problem, producing a failing test that reproduces the bug might be a robust first step. When the exam fails continually, you are able to target correcting the bug and view your examination go when the issue is settled. This technique makes certain that exactly the same bug doesn’t return Sooner or later.
To put it briefly, composing assessments turns debugging from the frustrating guessing recreation right into a structured and predictable system—assisting you catch far more bugs, speedier and more reliably.
Get Breaks
When debugging a difficult situation, it’s easy to become immersed in the trouble—watching your display screen for several hours, seeking solution following Remedy. But The most underrated debugging instruments is actually stepping absent. Getting breaks can help you reset your mind, decrease aggravation, and often see the issue from a new perspective.
When you're too close to the code for too long, cognitive exhaustion sets in. You might start overlooking apparent mistakes or misreading code that you simply wrote just hours earlier. In this point out, your Mind gets considerably less productive at difficulty-solving. A short wander, a espresso crack, as well as switching to a distinct activity for 10–quarter-hour can refresh your concentration. Quite a few developers report discovering the root of a dilemma once they've taken time for you to disconnect, letting their subconscious do the job from the history.
Breaks also enable avert burnout, Particularly during for a longer period debugging periods. Sitting before a display, mentally trapped, is not simply unproductive but additionally draining. Stepping absent lets you return with renewed Vitality as well as a clearer mindset. You may perhaps out of the blue notice a lacking semicolon, a logic flaw, or perhaps a misplaced variable that eluded you right before.
In case you’re stuck, a superb rule of thumb will be to set a timer—debug actively for forty five–60 minutes, then take a five–ten moment split. Use that point to move all over, stretch, or do a thing unrelated to code. It may sense counterintuitive, Particularly less than tight deadlines, but it surely really brings about quicker and more practical debugging Over time.
To put it briefly, taking breaks will not be an indication of weakness—it’s a wise strategy. It provides your Mind House to breathe, improves your point of view, and allows you avoid the tunnel eyesight That always blocks your progress. Debugging is often a psychological puzzle, and rest is a component of fixing it.
Master From Every Bug
Just about every bug you come upon is more than just A brief setback—It is really an opportunity to develop being a developer. Irrespective of whether it’s a syntax error, a logic flaw, or possibly a deep architectural difficulty, each one can teach you one thing worthwhile for those who take the time to reflect and evaluate what went Mistaken.
Start out by inquiring you a few important queries after the bug is settled: What prompted it? Why did it go unnoticed? Could it have been caught earlier with better practices like unit testing, code reviews, or logging? The answers often reveal blind places in the workflow or understanding and help you build stronger coding patterns going forward.
Documenting bugs can also be an excellent habit. Keep a developer journal or manage a log in which you Observe down bugs you’ve encountered, how you solved them, and Everything you discovered. As time passes, you’ll begin to see styles—recurring troubles or frequent blunders—that you could proactively avoid.
In workforce environments, sharing what you've acquired from the bug along with your peers is usually In particular effective. Whether or not it’s via a Slack concept, a short generate-up, or A fast understanding-sharing session, encouraging Some others stay away from the same challenge boosts group efficiency and cultivates a much better Finding out culture.
Additional importantly, viewing bugs as lessons shifts your mentality from stress to curiosity. Rather than dreading bugs, you’ll get started appreciating them as crucial parts of your progress journey. In the end, a lot of the greatest builders usually are not those who create great code, but people who continuously understand from their mistakes.
In the long run, each bug you correct provides a brand new layer on your skill set. So upcoming time you squash a bug, take a second to replicate—you’ll occur away a smarter, far more able developer due to it.
Summary
Improving upon your debugging abilities normally takes time, apply, and endurance — though the payoff is huge. It can make you a far more economical, confident, and capable developer. The subsequent time you might be knee-deep in a mysterious bug, bear in mind: debugging isn’t a chore — it’s a chance to be improved at what you do.